Your cart is currently empty!
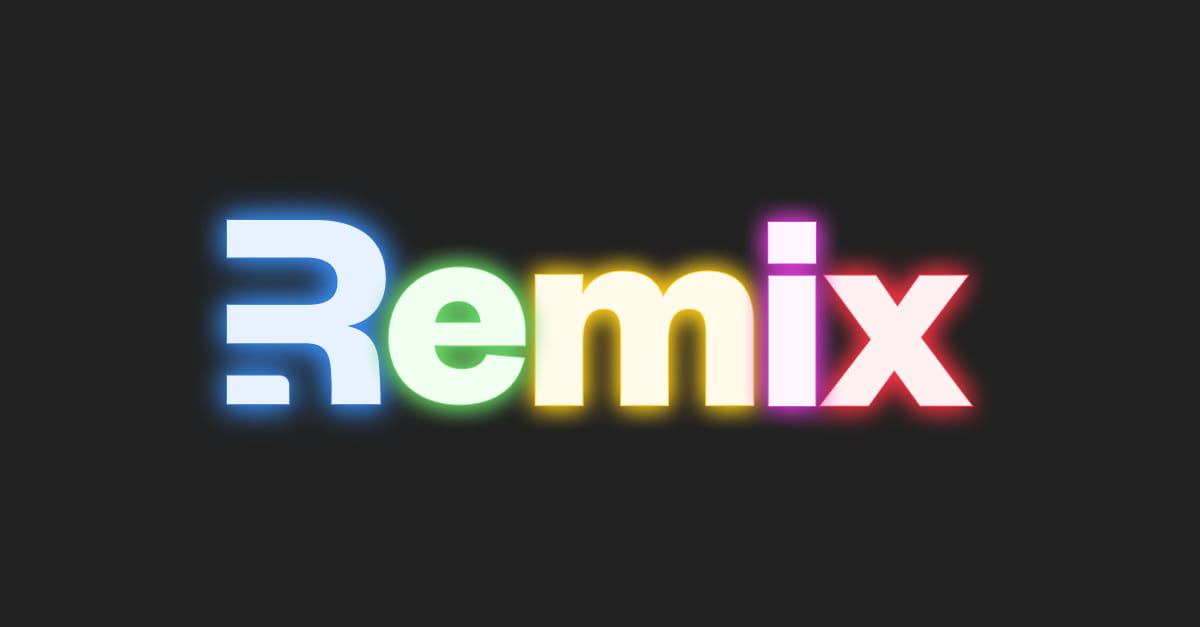
How Simple Error Handling in RemixJS V2!
This is a note to record REMIX Error Handling. CatchBoundary in REMIX v2 no longer exists, it becomes true of isRouteErrorResponse(useRouteError()).
https://remix.run/docs/en/main/route/error-boundary
https://remix.run/docs/en/main/start/v2#catchboundary-and-errorboundary
Structure
- return xxx: Default Page Components will be rendered.
- throw xxx: ErrorBoundary() will be rendered.
- throw json(): isRouteErrorResponse(error) is ‘true’ and will be rendered.
In REMIX V1, it uses CatchBoundary and ErrorBoundary to handle defined error and default error respectively. However, in V2, it simply becomes isRouteErrorResponse() hook.
BTW, I have a new post dedicated to my modern tech stack for Web constructing, please refer to 2024 React Remix 網頁開發流程。
Usage of ErrorBoundary().
This is used to get error infos, use const error = useRouteError();
1. throw json() in server-side (loader / action)
The isRouteErrorResponse(error) is ‘true’. You could call error.data, .status, .statusText, to get the things you contains.
2. throw xxx in server-side (loader / action)
Will renders error instanceof Error. Call what you throw in .message.
In order to handle Errors in REMIX, you could just add ErrorBoundary() in the route. It will be rendered when error occurred. You could put it in any route or the root, when error occurred, then the one in the route will call first, if it cannot find one, then the root will be called.
// root.tsx
import {
isRouteErrorResponse,
useRouteError
} from "@remix-run/react";
export default function App() {...}
export function ErrorBoundary() {
const error = useRouteError();
return (
<div>
{isRouteErrorResponse(error) ? (
<>
<h1>
{error.status} {error.statusText}
</h1>
<p>{error.data}</p>
</>
) : error instanceof Error ? (
<>
<h1>Error</h1>
<p>{error.message}</p>
<p>The stack trace is:</p>
<pre>{error.stack}</pre>
</>
) : (
<h1>Unknown Error</h1>
)}
</div>
);
}
Handling Page that Doesn’t Match Error
While V1 could handle the undefined routes, according to tutorial in V2, we could simply use the dynamic route! How simple and straight forward!
// ~/app/route/$any.tsx
import { Link } from "@remix-run/react";
// 404 page
export default function AnyPageNotMatch () {
return (
<div>
<h1>Page Not Found</h1>
<p>Sorry, that page does not exist.</p>
<Link to='/'>Back to Home</Link>
</div>
)
}